How to create a new contract
Starting with MetaGate version 1.18.0, the creation of contracts and the work with tokens are available in the #MetaHash network. #MetaHash token standard is analogous to ERC20 (Ethereum based token standard) but working on the TraceChain - the fastest blockchain in the world.
Work with contracts is carried out in the application: app: // MetaContracts
Creating a contract
Requirements:
- Version of the MetaGate is > 1.18.0;
- Wallet with the non-zero balance in the dev-network.
If all requirements are met, to create a new contract, go to the “Deploy new contract” app: // MetaContracts #! / AddContract /
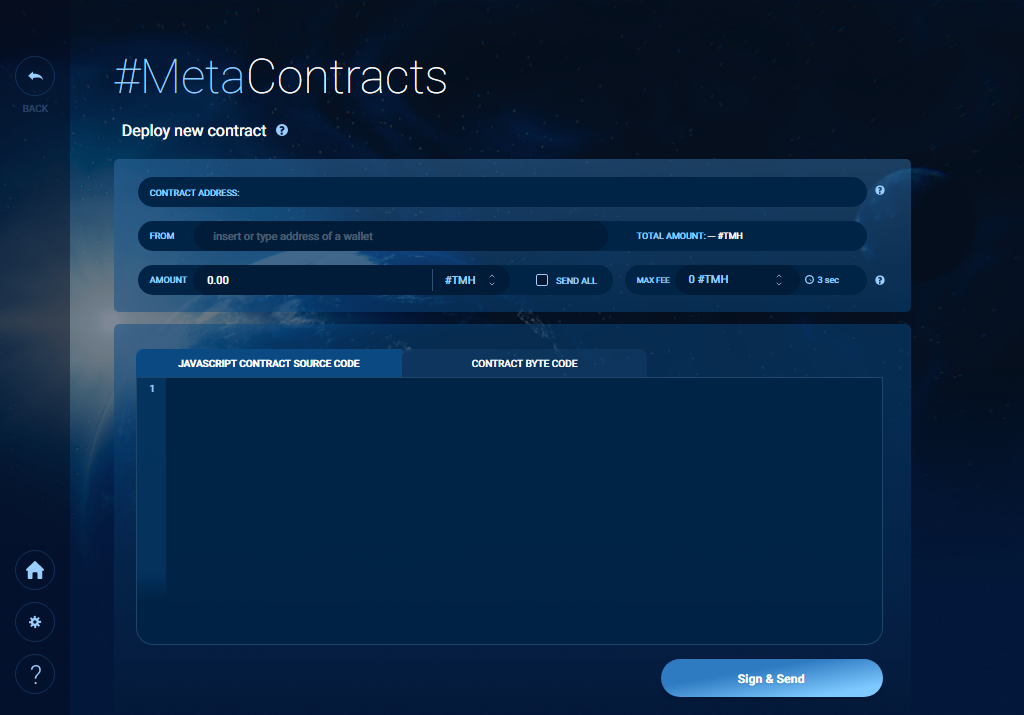
1.Deploy new contract
In the field “FROM” select wallet from the list, which has a non-zero balance in the dev-network.
After selecting a wallet, the contract address will automatically appear. And also the current balance of the wallet will be displayed in the “TOTAL AMOUNT” field.
You can specify the value of your token in the "AMOUNT" field, by default, the price of tokens is 0.00 TMH.
Contract constructor
After specifying the fields described above, it is necessary to describe the contract constructor the “JAVASCRIPT CONTRACT SOURCE CODE” field. In other words, the information which it consists.
For example, a constructor might be:
constructor() {
this.name = 'My_Contr';
this.owner = msg.sender;
this.ownerContract = null;
this.totalTokens = 0;
this.sum = 0;
this.balance = {};
}
Contract methods
After describing the constructor, it is necessary to describe the methods that you will need during the work with this contract - they are described there in the “JAVASCRIPT CONTRACT SOURCE CODE” field.
Please note: the methods are required, otherwise you will not be able to perform any actions in this contract. Moreover, the ability to add methods after saving the contract by editing it is not provided, only the methods described immediately when creating the contract will be available. If you need new methods, then you will have to create a new contract with a new list of methods.
WARNING: you can't edit the contract after the deployment.
Below is an example of a list of methods, it can be completely different at your discretion.
Initialization
Setting the address of the owner of the contract.
/* set contract owner */
setContract(_ownerContract) {
if (msg.sender == this.owner) {
this.ownerContract = _ownerContract;
}
}
Creating tokens within the contract
The method allows you to create tokens within the contract.
setOptions(tokenCreate) {
tokenCreate = parseInt(tokenCreate, 10) || 0;
/* set the amount, give the tokens to the contract */
if (msg.sender == this.ownerContract && this.sum == 0) {
this.totalTokens += tokenCreate;
if (this.balance[this.ownerContract] === undefined) {
this.balance[this.ownerContract] = 0;
}
this.balance[this.ownerContract] += tokenCreate;
} else {
throw new Error('No set tokens');
}
}
Conducting transactions with tokens
Transfer of the specified number of tokens from the address of the contract owner to any other address.
/* transfer tokens */
transfer(_to, _value) {
let addrSender = '';
addrSender = this.ownerContract;
_value = parseInt(_value, 10) || 0;
if (this.balance[addrSender] === undefined) {
this.balance[addrSender] = 0;
}
if (this.balance[_to] === undefined) {
this.balance[_to] = 0;
}
/* tokens are not enough */
if (this.balance[addrSender] < _value) {
throw new Error('tokens are not enough');
return false;
}
/* overflow */
if (this.balance[_to] + _value < this.balance[_to]) {
throw new Error('overflow');
return false;
}
this.balance[addrSender] -= _value;
this.balance[_to] += _value;
return true;
}
Thus, in this case, described as an example, the “JAVASCRIPT CONTRACT SOURCE CODE” field will contain the following information:
class Contract {
constructor() {
this.name = 'My_Contr';
this.owner = msg.sender;
this.ownerContract = null;
this.totalTokens = 0;
this.sum = 0;
this.balance = {};
}
/* set contract owner */
setContract(_ownerContract) {
if (msg.sender == this.owner) {
this.ownerContract = _ownerContract;
}
}
setOptions(tokenCreate) {
tokenCreate = parseInt(tokenCreate, 10) || 0;
/* set the amount, give the tokens to the contract */
if (msg.sender == this.ownerContract && this.sum == 0) {
this.totalTokens += tokenCreate;
if (this.balance[this.ownerContract] === undefined) {
this.balance[this.ownerContract] = 0;
}
this.balance[this.ownerContract] += tokenCreate;
} else {
throw new Error('No set tokens');
}
}
/* transfer tokens */
transfer(_to, _value) {
let addrSender = '';
_value = parseInt(_value, 10) || 0;
if (msg.sender == this.ownerContract) {
addrSender = this.ownerContract;
} else {
/* transfer between users*/
addrSender = msg.sender;
}
if (this.balance[addrSender] === undefined) {
this.balance[addrSender] = 0;
}
if (this.balance[_to] === undefined) {
this.balance[_to] = 0;
}
/* tokens are not enough */
if (this.balance[addrSender] < _value) {
throw new Error('tokens are not enough');
return false;
}
/* overflow */
if (this.balance[_to] + _value < this.balance[_to]) {
throw new Error('overflow');
return false;
}
this.balance[addrSender] -= _value;
this.balance[_to] += _value;
return true;
}
}
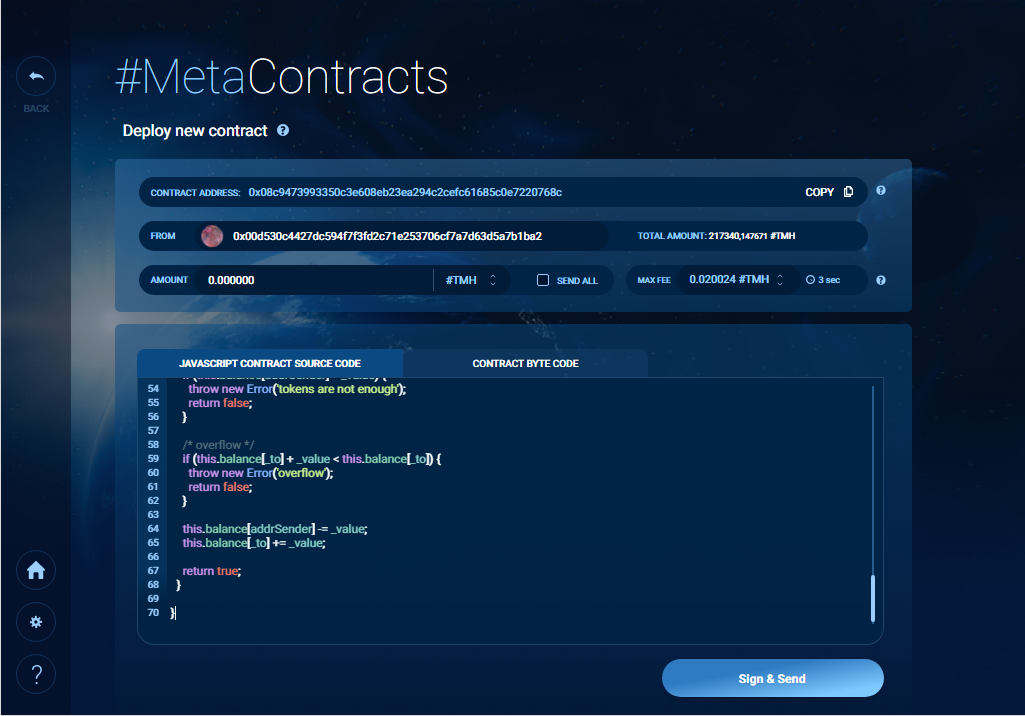
2.Creation of the contract
All the data entered during the creation of the contract is shown in picture 2. Next, you need to click on the “Sign & Send” button and enter the password of the wallet specified during the creation of the contract. As a result, the contract will be created.
Work with a contract
An example of a created contract is shown in picture 3.
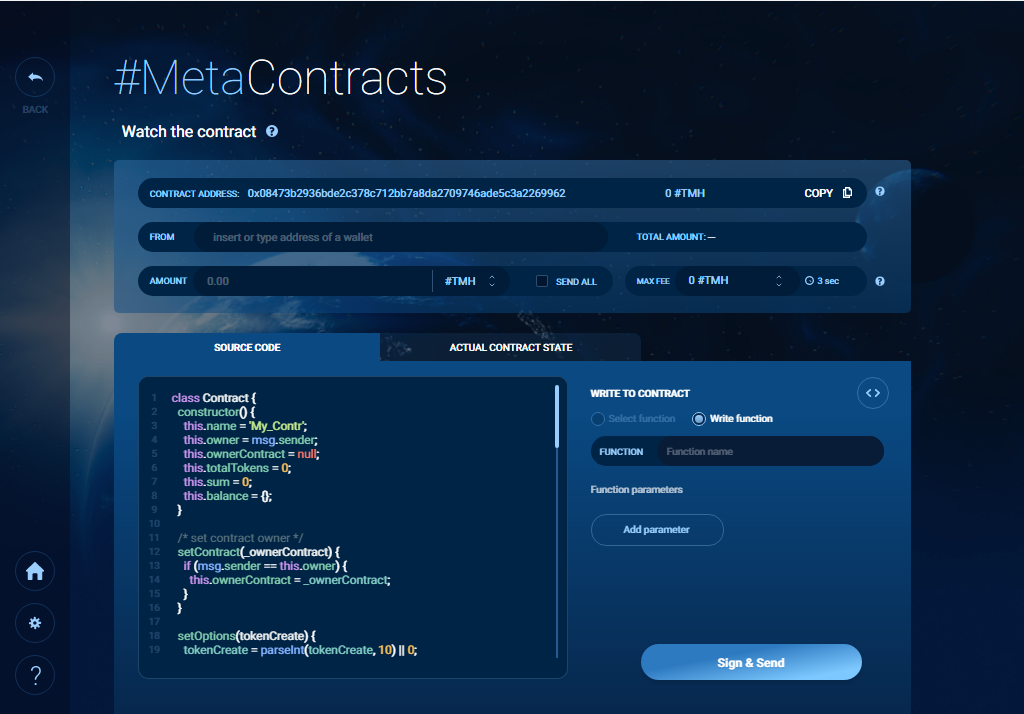
3.Example of a contract
In the “WRITE TO CONTRACT” section, you can apply all the methods described earlier. The “ACTUAL CONTRACT STATE” section displays the current status of the contract for all the fields that you described when you created the contract in its constructor.
Example of actions within the contract
- setContract
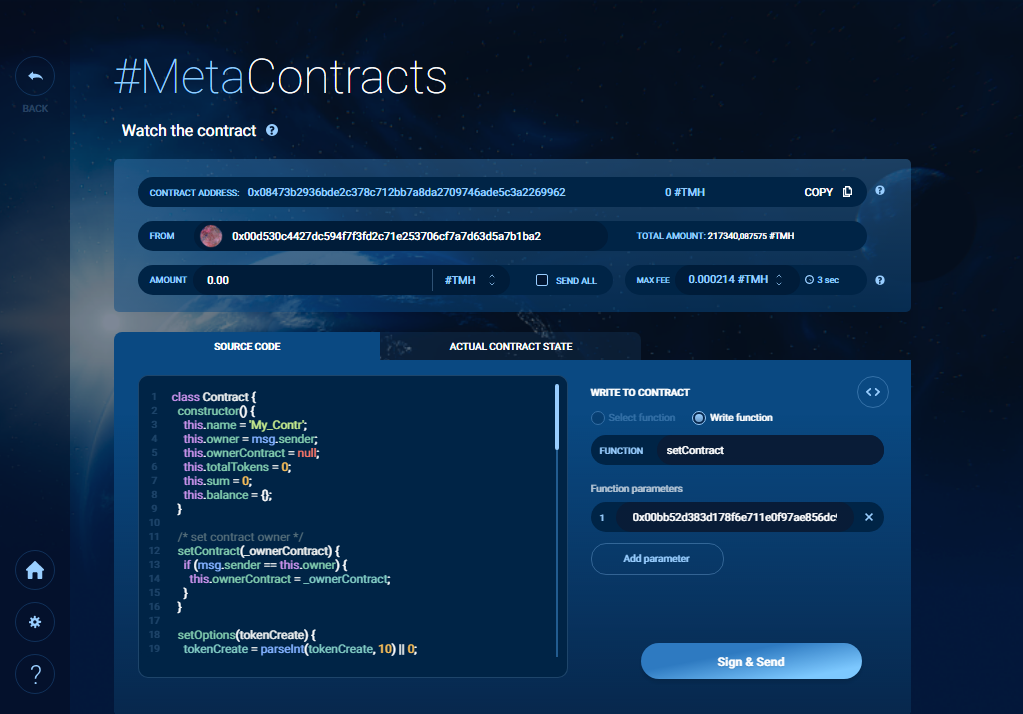
4.setContract
As a result, the selected wallet will become the owner of the contract.
- setOptions
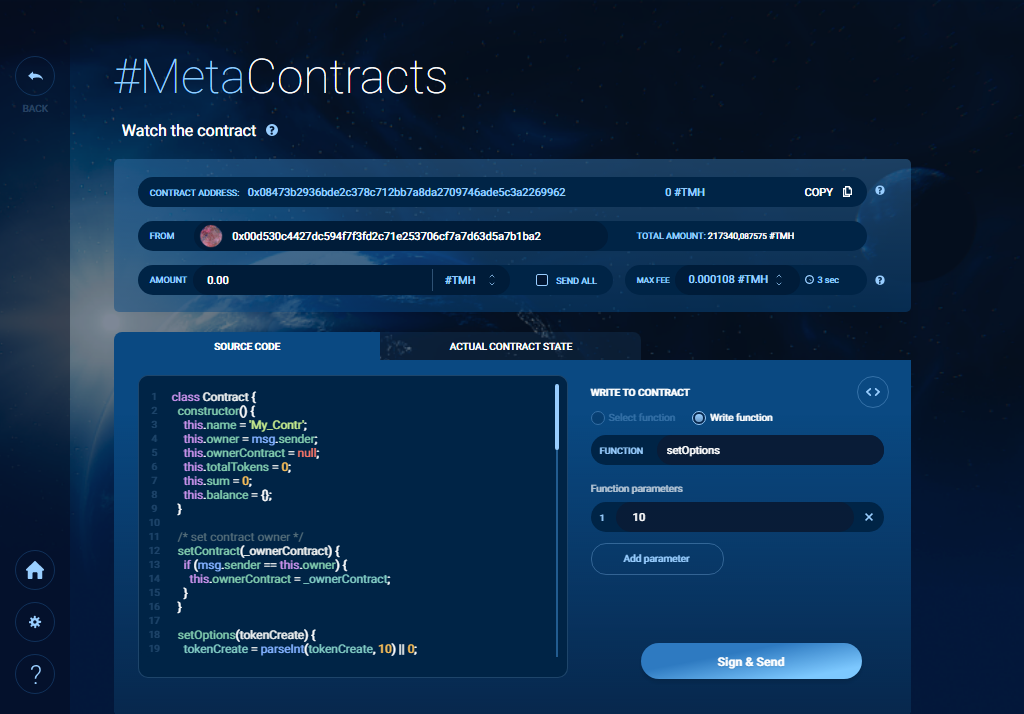
5.setOptions
As a result, 10 tokens will be created.
- transfers
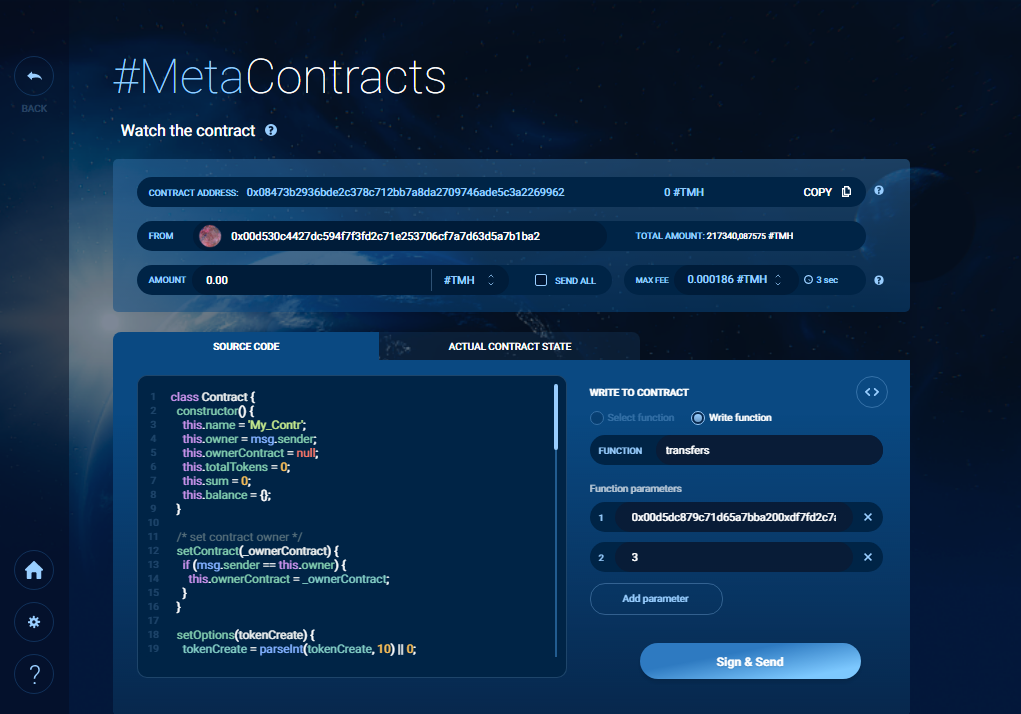
6.transfers
As a result, 3 tokens will be transferred to the specified address.
At any time in the section “ACTUAL CONTRACT STATE”, you can see the current status of the contract (picture 7).
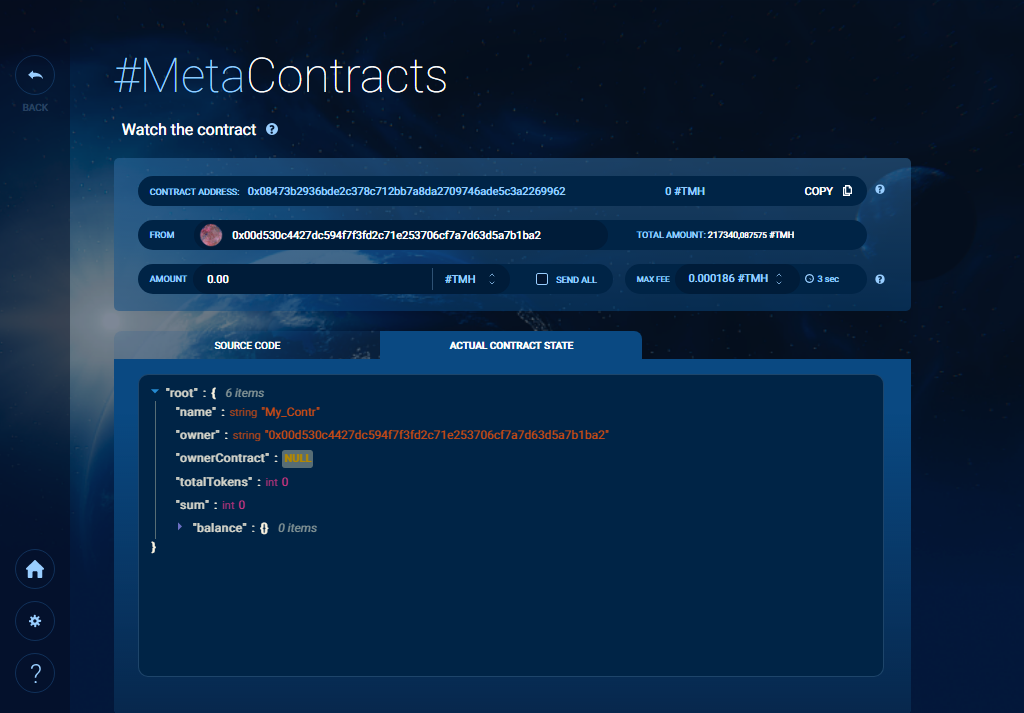
7.Actual contract state
Contract View Page
For your convenience, we have also made a page that allows you to view information on the contract: http://testpage.metahash.org/contract/
This page displays information on a single contract. The contract is taken as an example. The page displays information about all contract tokens, between which addresses and in what quantity they are distributed. Information is clearly presented in the form of a histogram.
You can view or download the source code of this page https://github.com/metahashorg/metahash-smart-example/tree/master/testpage_sourcecode and by analogy create pages for your contracts.
Updated over 6 years ago